Programming with Python | Chapter 1: Introduction to Programming & Python
Chapter Objectives
- Understand the fundamental concepts of programming.
- Learn what Python is and why it’s a popular programming language.
- Recognize the difference between high-level and low-level languages.
- Understand the role of an interpreter.
- Set up a Python development environment (Installation, REPL, IDE).
- Write and execute your first simple Python program.
Introduction
Welcome to the world of programming! This chapter introduces the foundational concepts you’ll need to begin your journey. We’ll explore what programming entails, discover the Python language and its strengths, and understand how computers execute instructions written in code. By the end of this chapter, you will have Python installed on your system and will have written and run your very first program. This marks the first step towards building powerful applications and solving complex problems with code.
Theory & Explanation
What is Programming?
At its core, programming is the process of writing instructions for a computer to follow. Computers, despite their apparent complexity, only understand very specific, low-level commands. Programming languages act as a bridge, allowing humans to write instructions in a more understandable format, which are then translated into a form the computer can execute. Think of it like writing a detailed recipe: each step must be precise and unambiguous for the desired outcome (the dish) to be achieved. Similarly, a program provides step-by-step instructions for the computer to perform a specific task, such as calculating a sum, displaying text, or controlling a robot.
flowchart TD A[Problem to Solve] --> B[Algorithm Development] B --> C[Code Writing] C --> D[Execution] D --> E[Testing & Debugging] E --> F{Works Correctly?} F -->|No| C F -->|Yes| G[Deployment/Use] style A fill:#f9d5e5,stroke:#333,stroke-width:1px style B fill:#d8e2dc,stroke:#333,stroke-width:1px style C fill:#ffe5d9,stroke:#333,stroke-width:1px style D fill:#ffcad4,stroke:#333,stroke-width:1px style E fill:#b8e0d2,stroke:#333,stroke-width:1px style F fill:#f1e3d3,stroke:#333,stroke-width:1px style G fill:#c7ceea,stroke:#333,stroke-width:1px
What is Python?
Python is a high-level, interpreted, general-purpose programming language. Let’s break down what that means:
- High-Level: Python uses syntax that is relatively close to human language (specifically English), abstracting away many of the complex details of the computer’s hardware. This makes it easier to read, write, and maintain code compared to low-level languages like Assembly or C.
- Analogy: Writing in Python is like giving driving directions using street names and landmarks (high-level), whereas writing in a low-level language is like specifying every single turn of the steering wheel and press of the pedals (low-level).
- Interpreted: Python code is executed line by line by an interpreter. This differs from compiled languages (like C++ or Java), where the entire source code is first translated into machine code (an executable file) before running. The interpreter reads your Python code and performs the actions directly.
- Analogy: An interpreter is like a human translator who translates a speech sentence by sentence as it’s being delivered. A compiler is like a translator who translates an entire book before it’s published.
- General-Purpose: Python isn’t designed for just one specific type of task. It’s versatile and used across various domains, including web development, data science, artificial intelligence, automation, scientific computing, and more.
Why Choose Python?
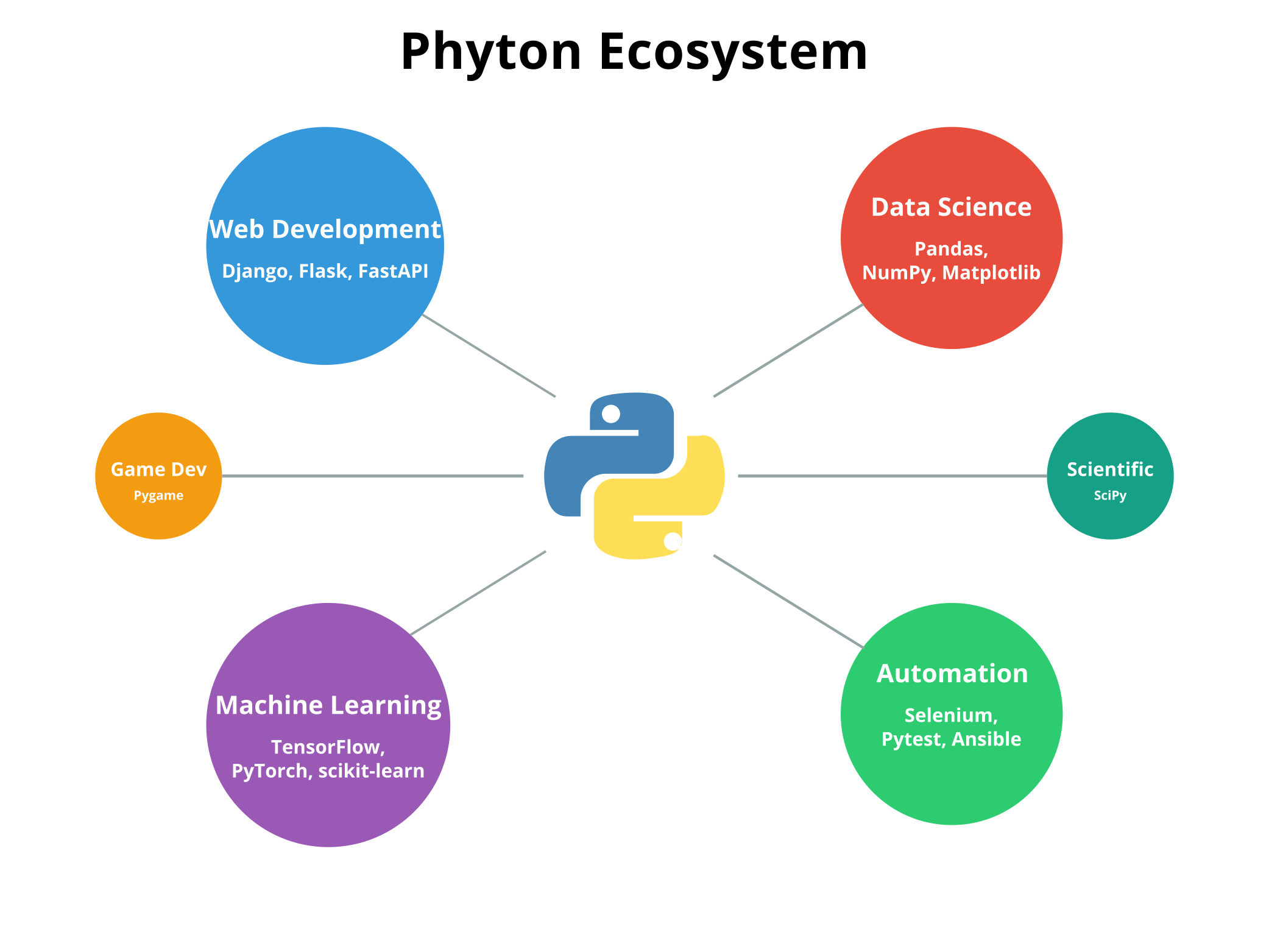
- Readability: Python’s syntax emphasizes clarity and readability, often resembling plain English.
- Simplicity: It’s relatively easy to learn, making it an excellent choice for beginners.
- Large Community & Libraries: Python boasts a massive, active community and a vast collection of pre-built code modules (libraries/packages) for almost any task, saving development time.
- Versatility: Applicable to a wide range of applications.
- Cross-Platform: Python code runs on various operating systems (Windows, macOS, Linux) without modification.
High-Level vs. Low-Level Languages
The Role of the Interpreter
The Python interpreter is a program that reads your Python code (.py
files) and executes it. It performs the following steps:
- Parsing: Reads the source code and checks for syntax errors.
- Compilation (to Bytecode): Translates the source code into an intermediate format called bytecode (
.pyc
files). Bytecode is a lower-level representation that’s platform-independent. - Execution: The Python Virtual Machine (PVM) executes the bytecode instructions.
graph TD A["Source Code (.py)"] --> B{"Python Interpreter"}; B -- "Parsing & Compiling" --> C["Bytecode (.pyc)"]; C --> D["Python Virtual Machine (PVM)"]; D -- "Execution" --> E["Output/Result"];
Setting Up Your Environment
To start writing and running Python code, you need:
- The Python Interpreter: Download and install Python from the official website: https://www.python.org/downloads/. Make sure to check the box “Add Python to PATH” during installation on Windows.
- A Code Editor or IDE (Integrated Development Environment): While you can write Python code in a simple text editor, using a code editor or IDE provides features like syntax highlighting, code completion, debugging tools, and project management, making development much easier. Popular choices include:
- VS Code (Visual Studio Code): Free, powerful, highly extensible (Recommended).
- PyCharm: Feature-rich IDE specifically for Python (Community Edition is free).
- Thonny: A beginner-friendly Python IDE.
- Sublime Text: A versatile text editor with Python support.
- The REPL (Read-Eval-Print Loop): Python comes with an interactive shell called the REPL. You can access it by typing
python
(orpython3
on some systems) in your terminal or command prompt. It allows you to type Python commands one at a time and see the results immediately. It’s great for quick tests and exploration.- Read: Reads the command you type.
- Eval: Evaluates (executes) the command.
- Print: Prints the result.
- Loop: Waits for the next command.
Code Examples
Example 1: Using the REPL
- Open your terminal or command prompt.
- Type
python
(orpython3
) and press Enter. You should see the Python prompt (>>>
). - Type the following command and press Enter:
print("Hello, Python World!")
Explanation:
- You’ll immediately see the output:
Hello, Python World!
print()
is a built-in Python function that displays output to the console."Hello, Python World!"
is a string literal – a sequence of characters enclosed in quotes. We’ll learn more about functions and strings in later chapters.
Example 2: Writing and Running a Python File
- Open your chosen code editor (e.g., VS Code).
- Create a new file named
hello.py
. - Type the following code into the file:
# hello.py
# This is a simple Python program that prints a greeting.
message = "Hello from a Python file!"
print(message)
print(5 + 3) # You can also print the result of calculations
Explanation:
#
denotes a comment. Comments are ignored by the interpreter and are used to explain the code to humans.message = "Hello from a Python file!"
assigns the string value to a variable namedmessage
. We’ll cover variables in detail in the next chapter.print(message)
displays the value stored in themessage
variable.print(5 + 3)
demonstrates thatprint()
can also display the result of expressions (in this case, the number 8).
Running the file:
- Save the
hello.py
file. - Open your terminal or command prompt.
- Navigate to the directory where you saved
hello.py
using thecd
(change directory) command. - Type
python hello.py
(orpython3 hello.py
) and press Enter.
You should see the following output:
Hello from a Python file!
8
Common Mistakes or Pitfalls
Forgetting print():
In.py
files, simply typing a value (like"Hello"
or5 + 3
) won’t display it. You need to use theprint()
function to see output. The REPL automatically prints the result of expressions, which can sometimes confuse beginners when moving to files.- Syntax Errors: Python is strict about syntax. A misplaced parenthesis, missing colon, or incorrect indentation will cause an error. Pay close attention to detail.
- Case Sensitivity: Python is case-sensitive.
message
is different fromMessage
orMESSAGE
. - Installation Issues (PATH): On Windows, forgetting to check “Add Python to PATH” during installation means the
python
command might not be recognized in the terminal. You may need to reinstall or manually configure the PATH environment variable.
Chapter Summary
- Programming involves writing instructions for computers using programming languages.
- Python is a high-level, interpreted, general-purpose language known for its readability and versatility.
- High-level languages abstract hardware details, while low-level languages are closer to machine code.
- An interpreter executes code line by line (often via bytecode).
- Setting up requires installing Python and choosing a code editor/IDE.
- The REPL (
>>>
) is useful for interactive testing. - Python code is typically saved in
.py
files and run from the terminal usingpython filename.py
. - The
print()
function is used to display output. - Comments (
#
) explain code; they are ignored by the interpreter.
Exercises & Mini Projects
Exercises
- REPL Exploration: Open the Python REPL and perform the following calculations:
15 * 4
100 / 5
2 ** 5
(2 to the power of 5)- Use
print()
to display the text “Learning Python is fun!”.
- File Execution: Create a file named
info.py
. Inside it, use theprint()
function multiple times to display your name, your favorite hobby, and the current year on separate lines. Run the file from the terminal. - Syntax Error Practice: Create a file named
error.py
. Intentionally introduce a syntax error (e.g.,print("Hello"
without the closing parenthesis). Run the file and observe the error message Python provides. Try to understand what the message means. Correct the error and run it again. - Comments: Write a short Python program (e.g., one that prints two different messages) and add comments explaining what each line does.
Mini Project: Simple Greeting Generator
Goal: Create a Python script greeting.py
that asks the user for their name and then prints a personalized greeting.
Steps:
- Use the
input()
function to ask the user for their name. Theinput()
function pauses the program and waits for the user to type something and press Enter. It returns what the user typed as a string.- Example:
user_name = input("Please enter your name: ")
- Example:
- Store the user’s input in a variable (e.g.,
user_name
). - Use the
print()
function to display a greeting that includes the user’s name. You can combine strings using the+
operator (string concatenation).- Example:
print("Hello, " + user_name + "! Welcome to Python.")
- Example:
- Save the file as
greeting.py
and run it from the terminal. Test it by entering your name when prompted.
(Hint: We haven’t formally covered input()
or variables yet, but try using the examples above to figure it out! This encourages exploration.)
Additional Sources: